In this latest post, we will discuss what are conditional statements in JavaScript. These statements are a bit confusing when coding, we'll learn this in a very simple way.
JavaScript supports conditional statements which are used to perform different actions based on different conditions.
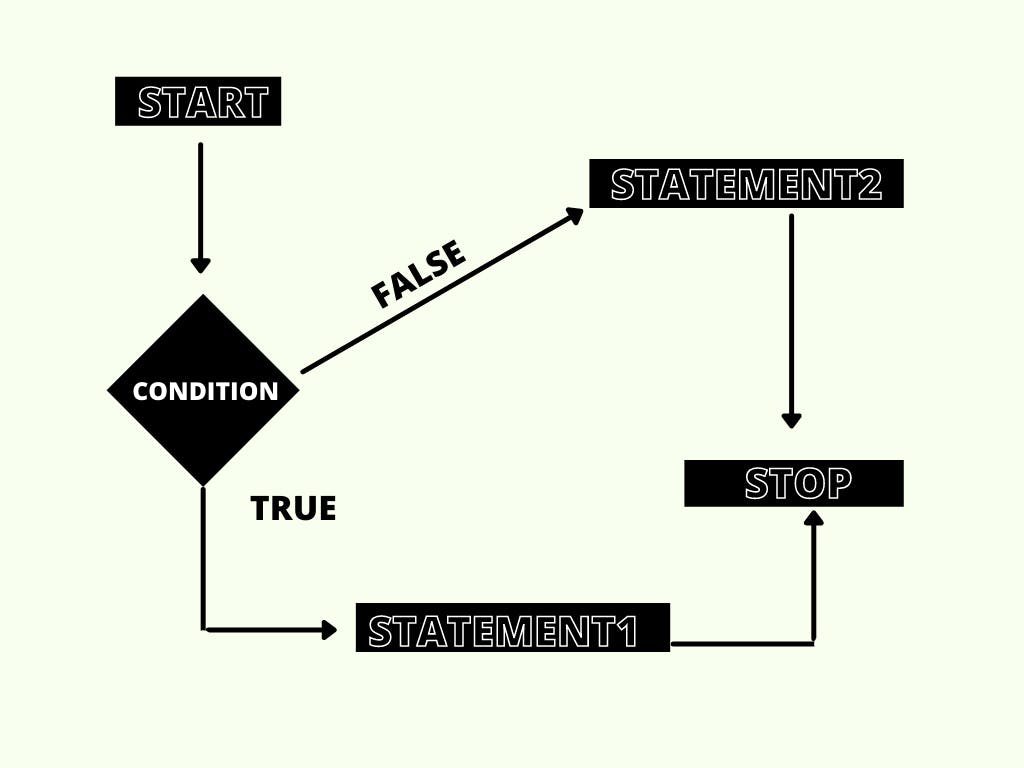
Two types of Conditonal Statements:
-
if...else
(Has various forms)-
if
statement -
if...else
statement -
if...else if
statement
-
-
switch...case
General syntax for if...else
statement:
if (condition1) {
statement
} else if {
statement2
} else {
statement3
}
The if a statement executes a statement if a specified condition is true. If the condition is false, another statement can be executed.
-
if
statements: if a condition is true, it executes a specific block of code. -
else
statements: if the same condition is false, it executes a specific block of code. -
else if
: this specifies a new test if the first condition is false.
EXAMPLE: To check if a number is positive or, negative or zero.
if (a > 0) {
var result = "positive";
} else if (a === 0 ) {
var result = "zero";
} else {
var result = "negative";
}
// If a = 10 returns positive
// if a = 0 returns zero
// if a = -9 returns negative
Switch case
(Src: FCC) A switch statement tests a value and can have many case statements which define various possible values. Statements are executed from the first matched case value until a break is encountered.
switch(expression) {
case x:
// code block
break;
case y:
// code block
break;
default:
// code block
}
- expression: An expression whose result is matched against each case clause
- case valueN (optional): A case clause used to match against expression. If the expression matches the specified valueN, the statements inside the case clause are executed until either the end of the switch statement or a break.
- default: A default clause; if provided, this clause is executed if the value of expression doesn't match any of the case clauses.
EXAMPLE:
function name(val) {
var answer = "";
switch(val) {
case 1:
return "Rahul";
break;
case 2:
return "Sam";
break;
case 3:
return "John";
break;
case 4:
return "Chris";
break;
}
return answer;
}
name(1);
// if val = 1, returns "Rahul".
// if val = 2, returns "Sam",
// if val is empty then returns ""
Simple to understand!
Originally -> https://rahulism.tech/article/conditional-statements-in-javascript/
😊Thanks For Reading | Happy Coding 😁
Comments (0)