As a JavaScript application begins to grow, it becomes harder and harder to organize and manage. It is therefore advisable to cut it into small functional pieces that are easier to handle and whose stewardship is less problematic.
Beyond the division into functions and classes one can also cut the code into coherent modules having a certain level of abstraction. On the other hand a module embarks its stewardship and becomes easy to use and reuse. Unfortunately, JavaScript does not know the namespaces that exist in multiple languages.
With ES5 you can get by with objects and closures and there are a multitude of implementations. ES6 finally offers us a native modularization!
ES6 modules
The ES6 modules:
- have a simple syntax and are based on the division into files (a module = a file),
- are automatically in "strict" mode,
- provide support for asynchronous loading.
Modules must expose their variables and methods explicitly. So we have two keywords:
- export: to export everything that must be accessible outside the module,
- import: to import everything that must be used in the module (and that is exported by another module).
Export and import
Export
Since everything written in a module is internal to it, you have to export what you want to make usable by the other modules.
To export, use the export keyword:
export function rename(name) {
var person = new Person();
return person.changeNom(name);
}
export class Identite {
// code
}
function verify() {
// code
}
Here we export the rename method and the Identite class, but the verify method remains internal to the module.
Another way to do this is to group what needs to be exported:
function rename(name) {
var person = new Person();
return person.changeNom(name);
}
class Identite {
// code
}
function verify() {
// code
}
export { rename, Identite };
Import
To import into a module something that is exported by another module we use the keyword import:
import { rename, Identite } from "./identite.js";
//We can also rename what we export with the same syntax.
import { rename as renameIdentite, Identite } from "./identite.js";
//If we want to import everything from a module we can use this syntax:
import * from "./identite.js";
Loading modules
We saw above that the syntax is simple. What is less is that currently it is not recognized by our browsers and must therefore manage to operate all that.
On the other hand, ES6 does not specify how modules should be loaded, in other words the implementation of this aspect is left to the discretion of developers, probably because it was difficult to define a universal specification.
In addition to the development of the client aspect of an application (the frontend) we do not only have JavaScript, there is necessarily also CSS code with probable use of a Sass type preprocessor. It would be nice to have a tool that allows us to manage all that. The good news is that it exists!
There are even several solutions but the one that seems to have the most success is Webpack. It is given modules with dependencies (js, css, png ...) and it transforms all this into usable static assets:
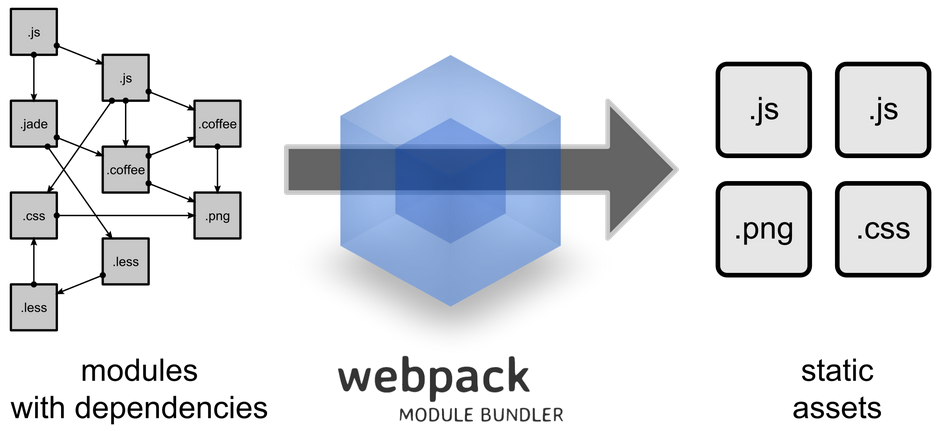
Webpack
Installation
To use Webpack you must first install it. But you must already have:
node.js, so install it if you do not have it, it will serve you for many other things!
npm, it's the dependency manager of node.js, you also have to install it if you do not have it (the good news is that it installs with node.js).
You can then install Webpack:
npm install webpack -g
Then you have to initialize npm:
npm init
Accept all defaults (they are only of interest if you want to publish a package), so you create a package.json file like this:
{
"name": "test",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC"
}
You are now ready to install items in your project. We will add Babel which will allow us to transform the code ES6 into ES5 code (I also install Webpack in the project):
npm install babel-core babel-loader babel-preset-es2015 webpack --save-dev
It takes a little while for the node_modules folder to be created and filled with all the dependencies.
If you look at your package.json file you will find these added dependencies:
"devDependencies": {
"babel-core": "^6.26.0",
"babel-loader": "^7.1.2",
"babel-preset-es2015": "^6.24.1",
"webpack": "^3.10.0"
}
Comments (0)