Bash is a Unix shell, which is a command line interface (CLI) for interacting with an operating system. Any command that you can run from the command line can be used in a bash script. Scripts are used to run a series of commands. Bash is available by default on Linux and macOS operating systems.
Let's have a hypothetical scenario where you need to execute a BASH script on multiple remote servers, but you don't want to manually copy the script to each server, then again login to each server individually and only then execute the script.
There's a better way to do that and it would save you the first two steps.
Prerequisites
- I've created a really simple BASH scrip that we will use for our example
- I've deployed 3 servers on Digital Ocean that we would use
The BASH Script
This is the demo script that I've prepared. It simply executes a few checks like the current memory usage, the current CPU usage, the number of TCP connections and the version of the kernel.
Copy the code bellow and add this in a file called remote_check.sh
#!/bin/bash
##
# BASH script that checks the following:
# - Memory usage
# - CPU load
# - Number of TCP connections
# - Kernel version
##
##
# Memory check
##
server_name=$(hostname)
function memory_check() {
echo "#######"
echo "The current memory usage on ${server_name} is: "
free -h
echo "#######"
}
function cpu_check() {
echo "#######"
echo "The current CPU load on ${server_name} is: "
echo ""
uptime
echo "#######"
}
function tcp_check() {
echo "#######"
echo "Total TCP connections on ${server_name}: "
echo ""
cat /proc/net/tcp | wc -l
echo "#######"
}
function kernel_check() {
echo "#######"
echo "The exact Kernel version on ${server_name} is: "
echo ""
uname -r
echo "#######"
}
function all_checks() {
memory_check
cpu_check
tcp_check
kernel_check
}
all_checks
Digital Ocean Droplets
If you don't have a Digital Ocean account yet, you can sign up for Digital Ocean and get $50 free credit via this link here:
Once you have your Digital Ocean account ready go ahead and deploy 3 droplets.
I've gone ahead and created 3 Ubuntu servers:
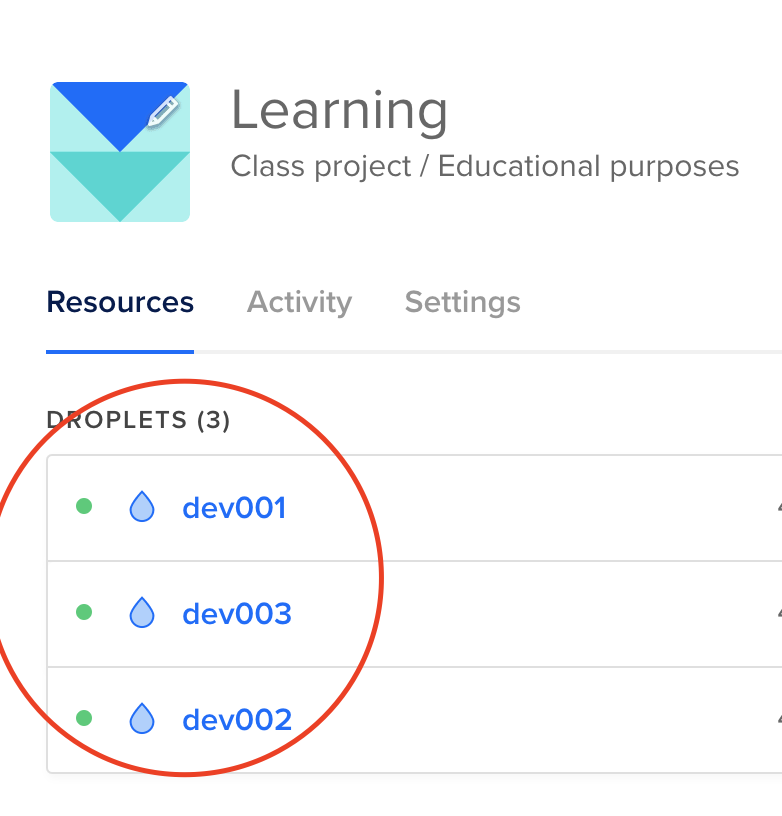
I'll put a those servers IP's in a servers.txt file which I would use to loop though.
Running the Script on all Servers
Now that we have the script and the servers ready and that we've added those servers in our servers.txt file we can run the following command to loop though all servers and execute the script remotely without having to copy the script to each server and individually connect to each server.
for server in $(cat servers.txt) ; do ssh root@${server} 'bash -s' < ./remote_check.sh ; done
What this for loop does is, it goes through each server in the servers.txt file and then it runs the following command for each item in the list:
ssh root@the_server_ip 'bash -s' < ./remote_check.sh
You would get the following output:
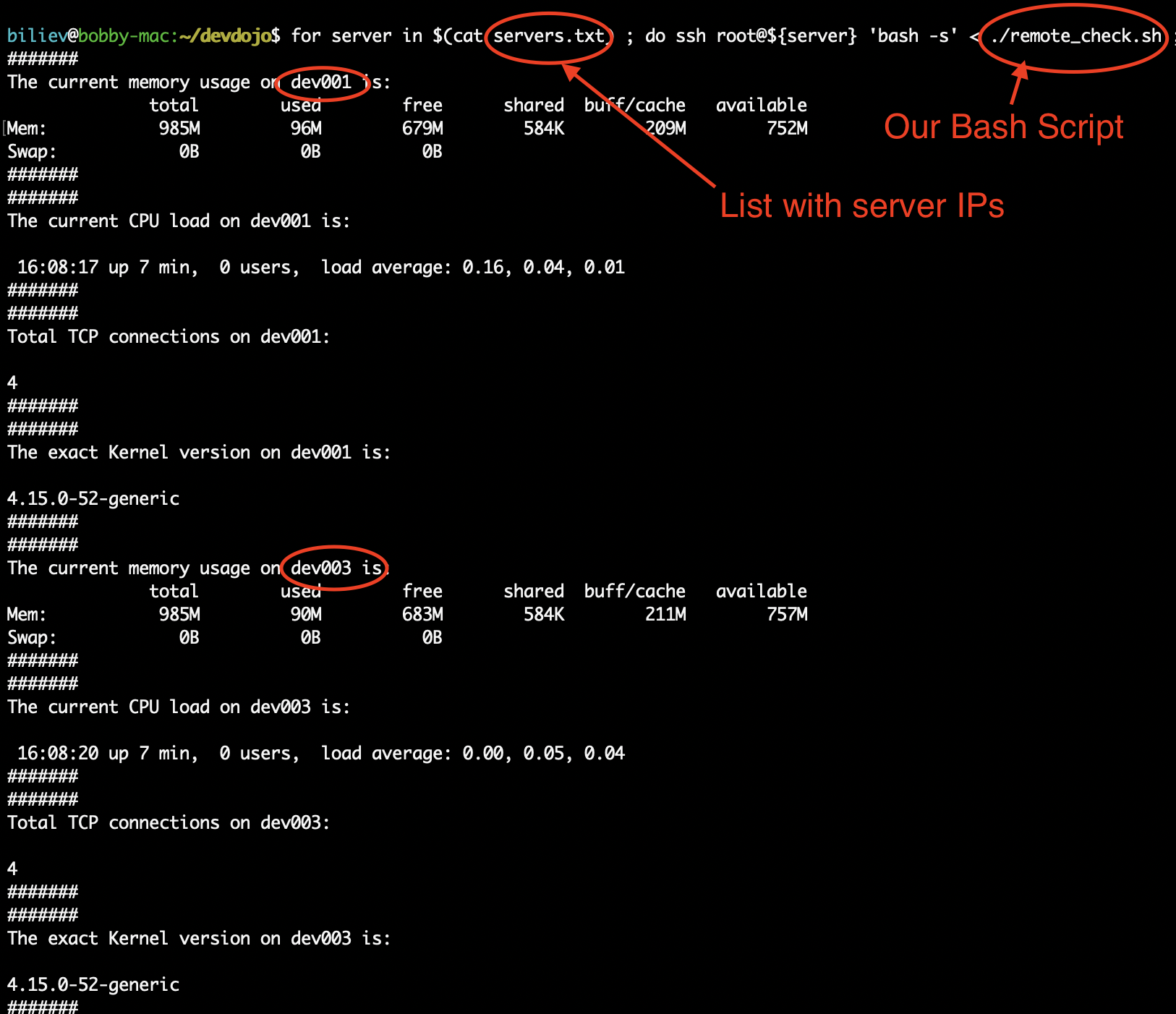
Conclusion
This is just a really simple example on how to execute a simple script on multiple servers without having to copy the script to each server and without having to access the servers individually.
Of course you could run a much more complex script and on many more servers.
Hope that this helps and make sure to reach out to me in case that you have any questions!
Comments (0)