🤓 Code Snippets
Share and store your most common code snippets. Use our snippet explanation generator to use AI to try and explain the code.
fn main() {
let error: Result<(), &str> = Err("error"); // Error
// Show error instead of panic
match error {
Ok(_) => println!("Ok"),
Err(e) => println!("Error: {}", e),
}
println!("Still running 🍕");
// Output:
// Error: error
// Still running 🍕
}
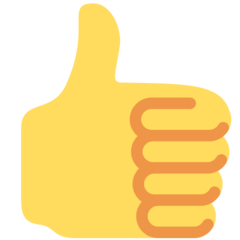
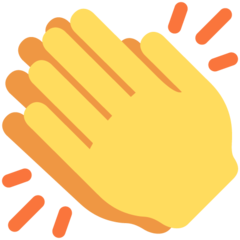
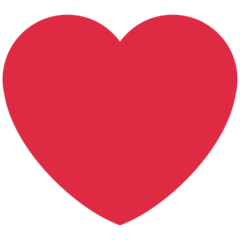
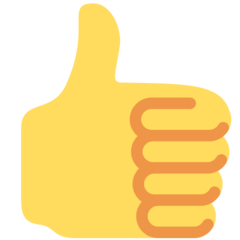
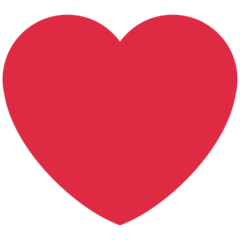
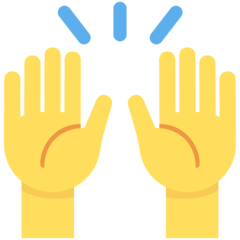
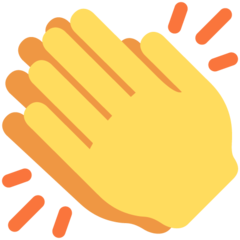
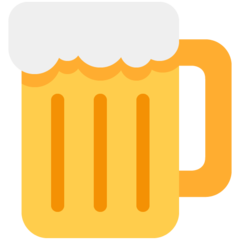
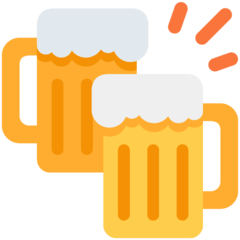
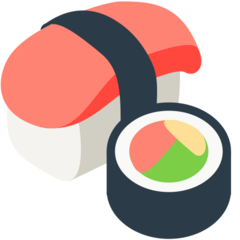
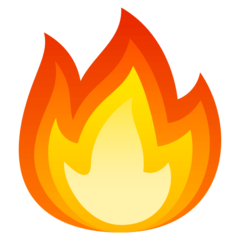
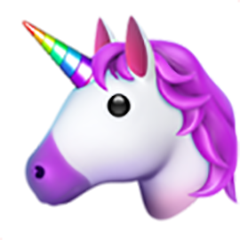
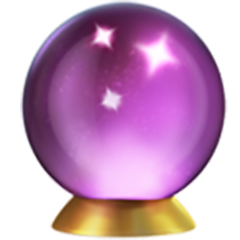
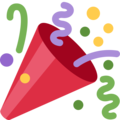
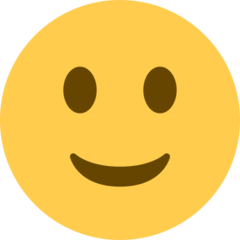
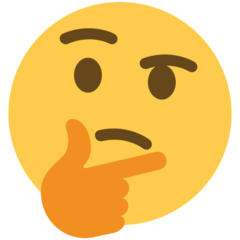
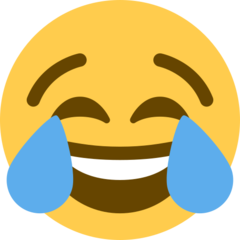
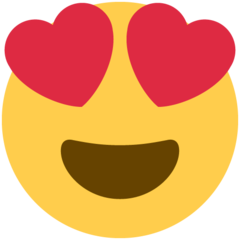
<?php
return [
'cache' => false,
/*
|--------------------------------------------------------------------------
| View Storage Paths
|--------------------------------------------------------------------------
|
| Most templating systems load templates from disk. Here you may specify
| an array of paths that should be checked for your views. Of course
| the usual Laravel view path has already been registered for you.
|
*/
'paths' => [
resource_path('views'),
],
/*
|--------------------------------------------------------------------------
| Compiled View Path
|--------------------------------------------------------------------------
|
| This option determines where all the compiled Blade templates will be
| stored for your application. Typically, this is within the storage
| directory. However, as usual, you are free to change this value.
|
*/
'compiled' => env(
'VIEW_COMPILED_PATH',
realpath(storage_path('framework/views'))
),
];
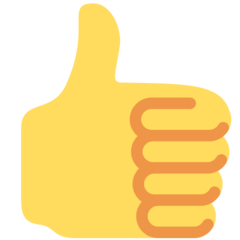
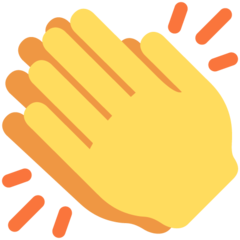
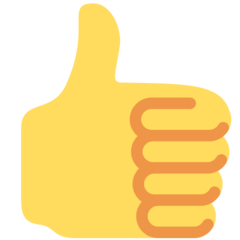
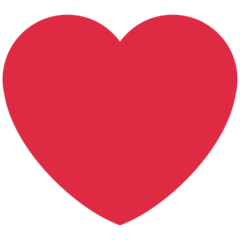
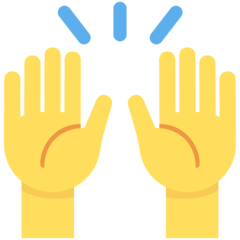
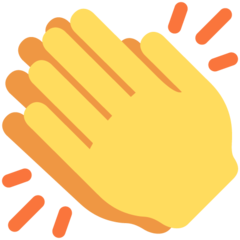
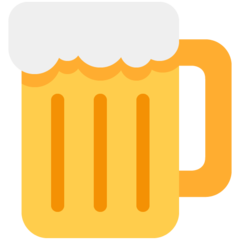
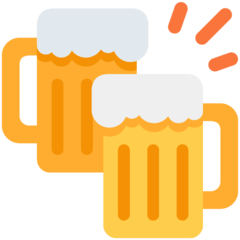
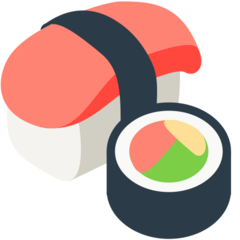
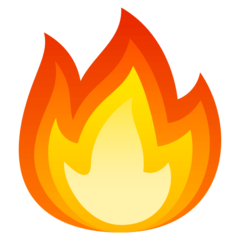
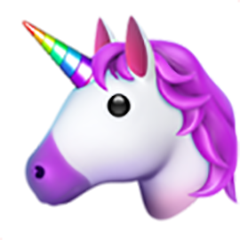
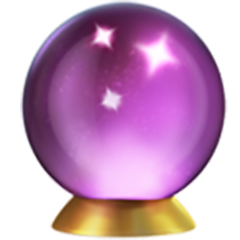
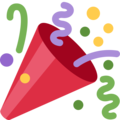
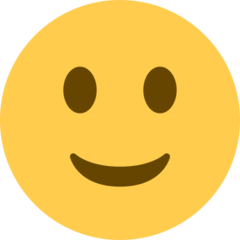
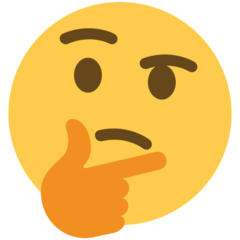
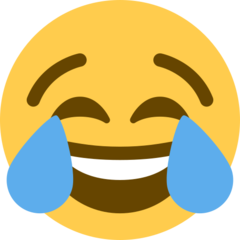
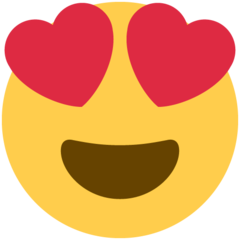
<?php
public function multiply($a, $b) {
return $a * $b;
}
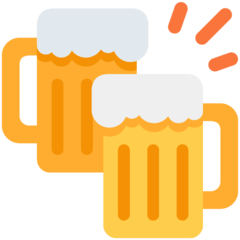
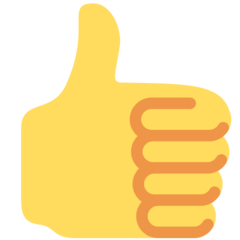
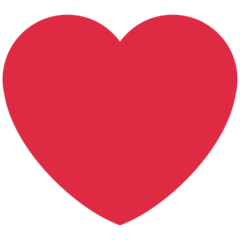
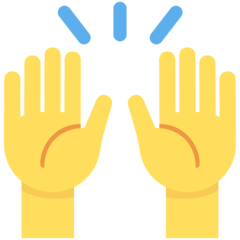
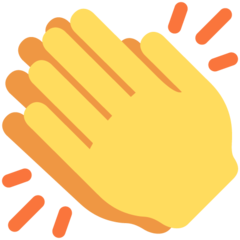
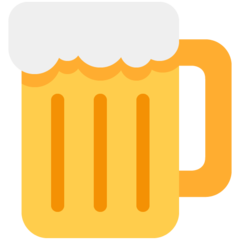
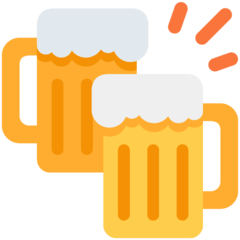
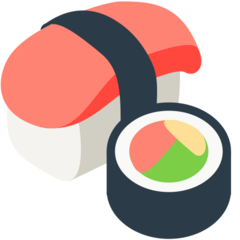
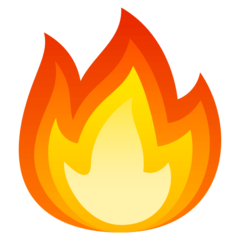
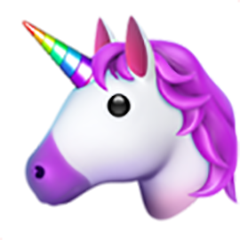
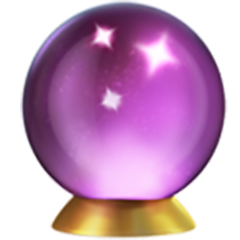
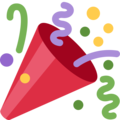
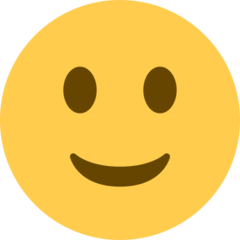
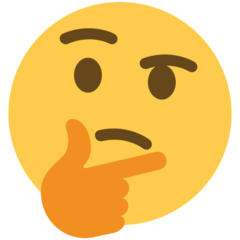
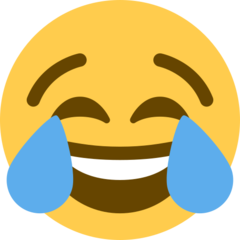
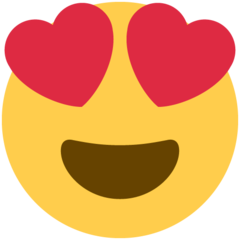
git diff --name-only --diff-filter=U
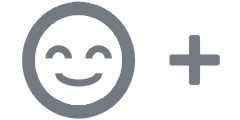
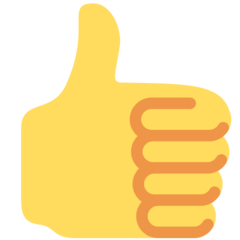
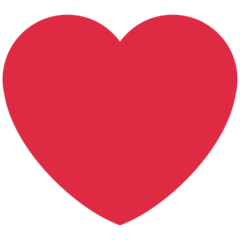
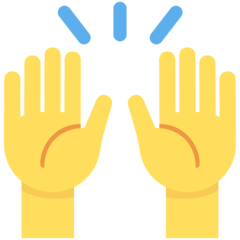
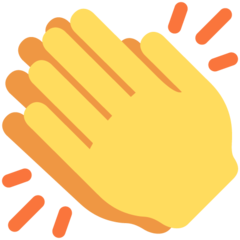
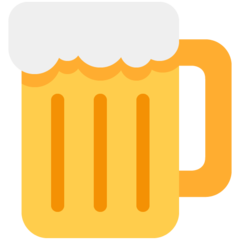
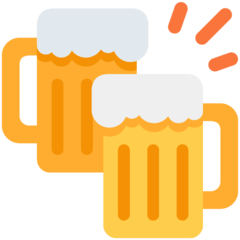
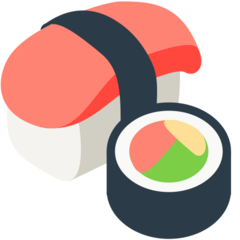
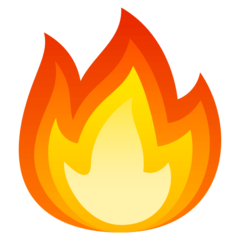
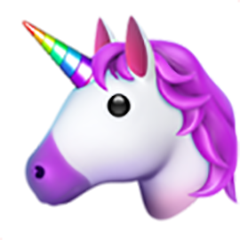
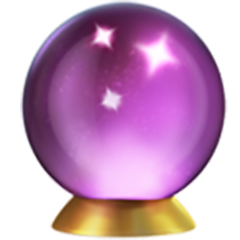
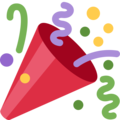
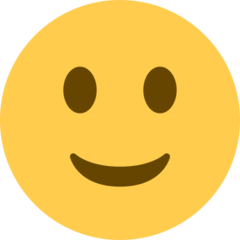
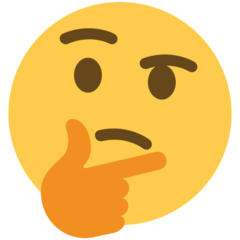
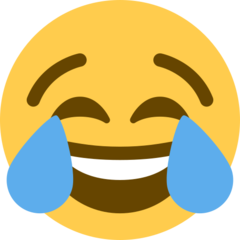
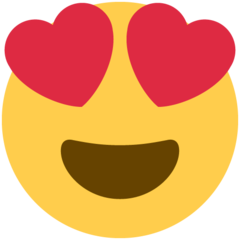
Form validation with class-validator
import { validateOrReject, Length, IsEmail, IsString} from "class-validator";
import type { ValidationError } from "class-validator";
// validation
export class LoginValidation {
@IsEmail()
email!: string | null;
@IsString()
@Length(8, 16)
password!: string | null;
}
export async function validateInput (input: LoginValidation) {
try {
await validateOrReject(input);
return { errors: {} };
} catch (err) {
console.warn("[Validations] error");
const validationErrors = err as ValidationError[];
const errorsList: Record<string, string> = validationErrors.reduce(
(prevError, currError) => {
const property = currError.property;
const message = Object.values(currError.constraints!)[0];
return {...prevError, [property]: message };
},{});
return { errors: errorsList };
}
}
//example
const validation = new LoginValidation();
validation.email = "email"
validation.password="some+difficult-password"
const validate = await validateInput(validation);
//{errors: {email: "email must be a valid email"}}
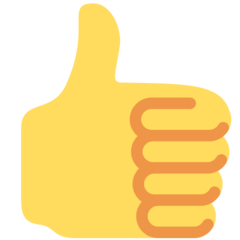
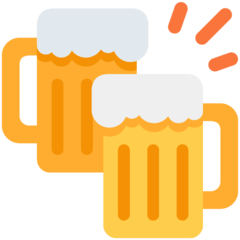
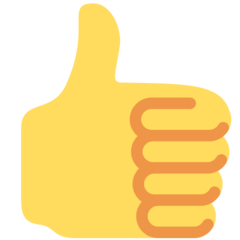
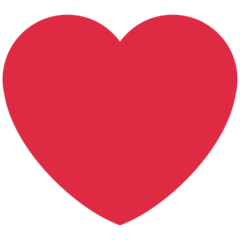
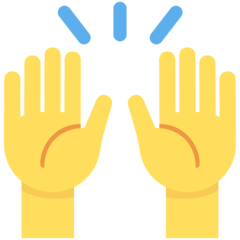
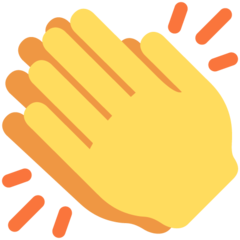
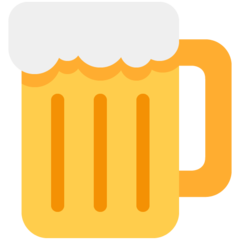
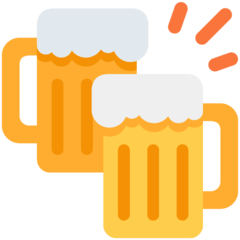
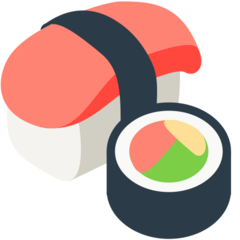
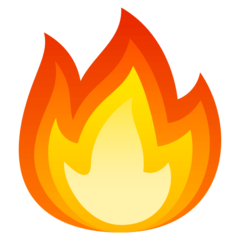
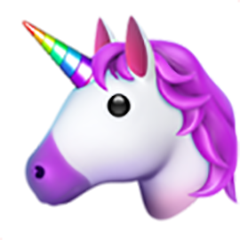
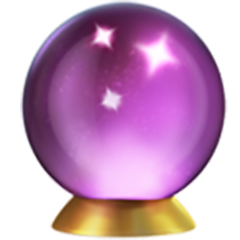
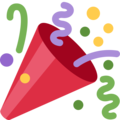
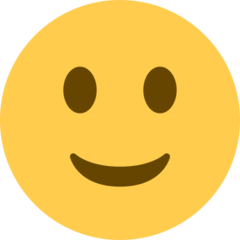
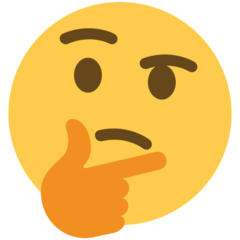
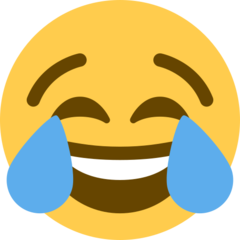
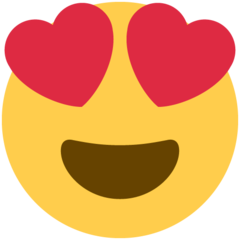
from flask import Flask
app = Flask(__name__)
@app.route('/', methods = ['GET'])
def root():
message = 'Hello, World!'
return message
if __name__ == '__main__':
app.run(host='127.0.0.1', port=5000, debug=False)
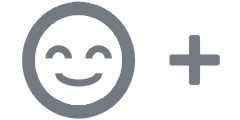
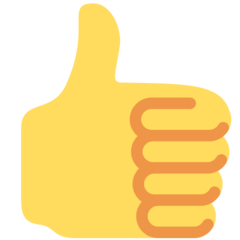
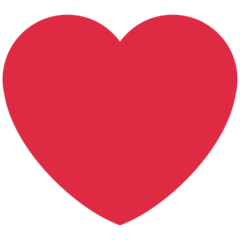
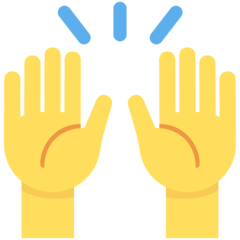
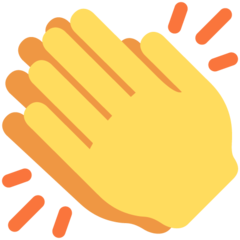
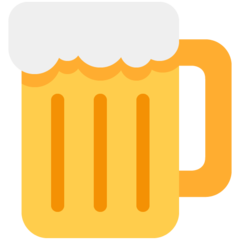
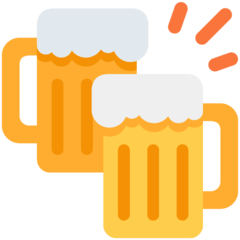
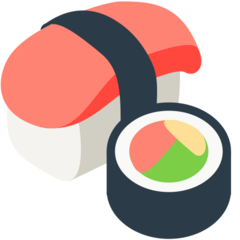
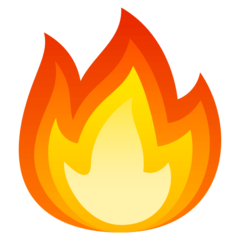
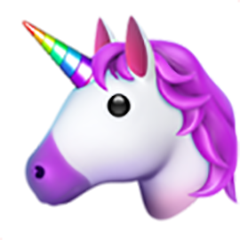
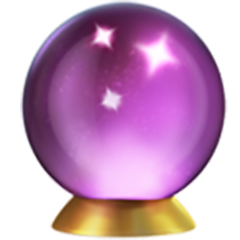
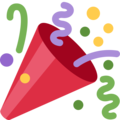
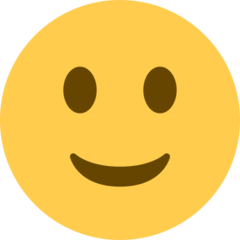
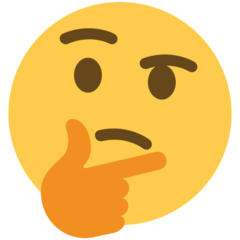
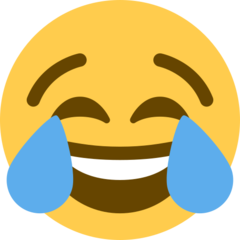
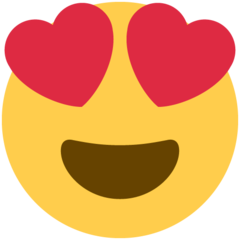
const rand = () => {
return Math.random().toString(36).substr(2);
};
const token = () => {
return rand() + rand();
};
console.log(token());
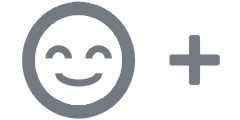
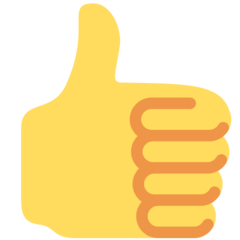
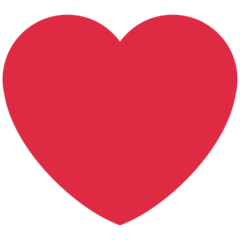
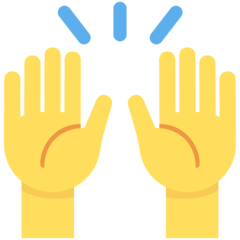
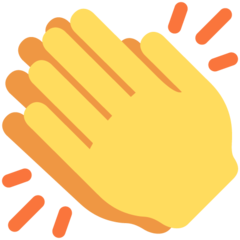
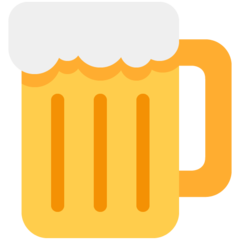
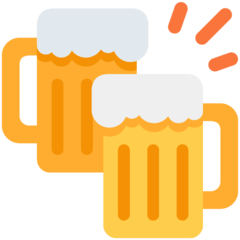
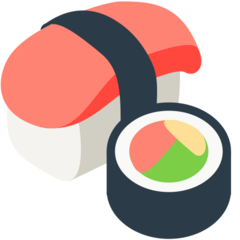
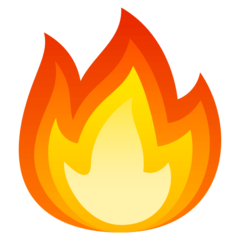
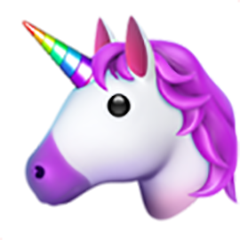
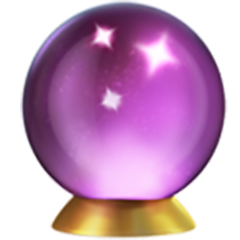
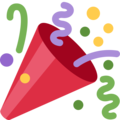
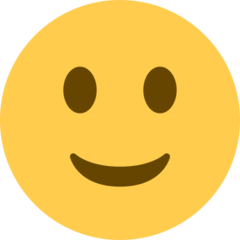
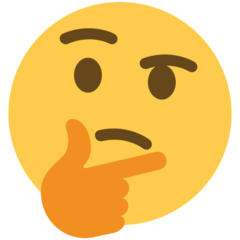
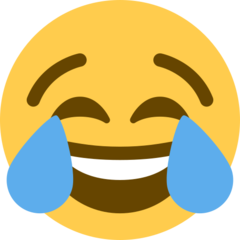
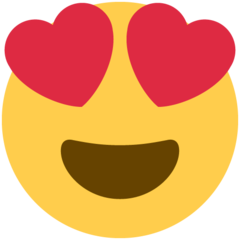
const random = Math.floor(Math.random() * 9000 + 1000);
console.log(random);
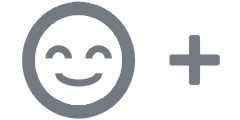
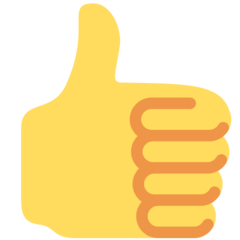
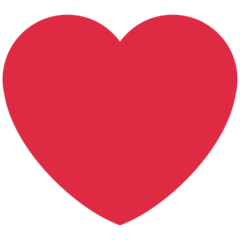
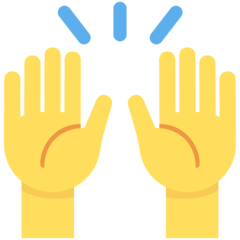
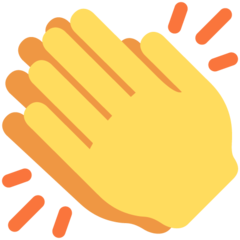
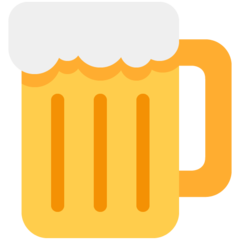
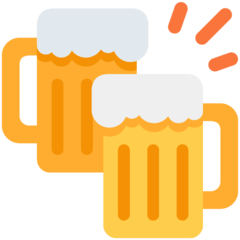
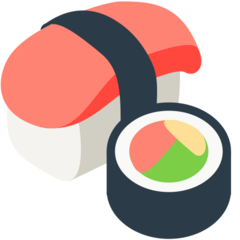
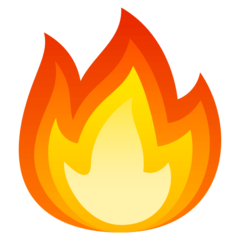
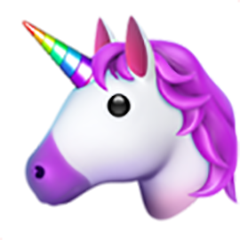
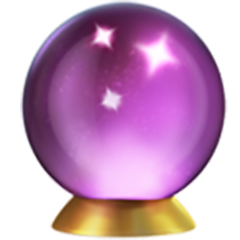
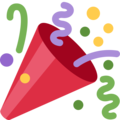
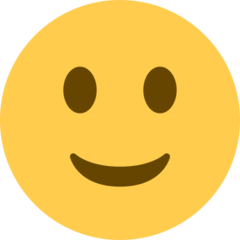
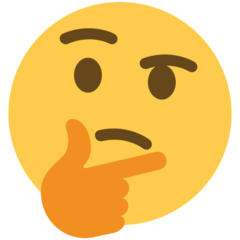
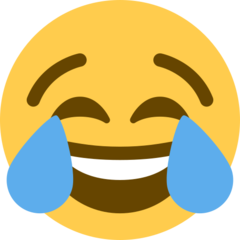
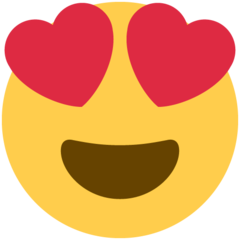
Funny opensource commit
while True:
github.commit(target: opensource)
print("YAY! New commit to open source!")
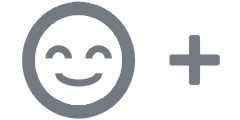
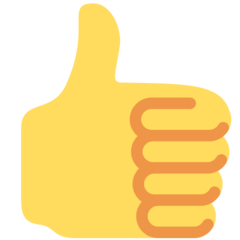
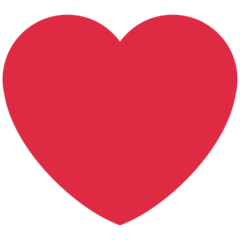
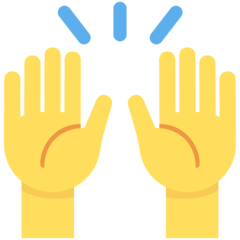
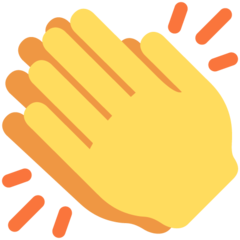
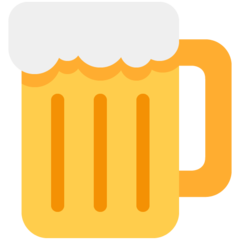
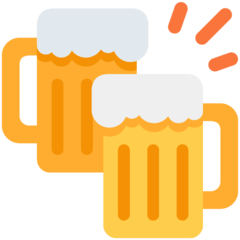
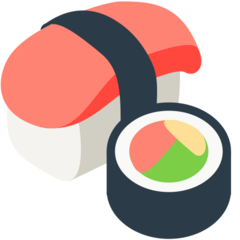
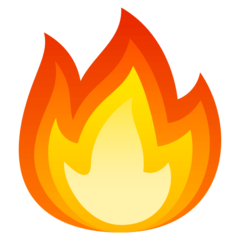
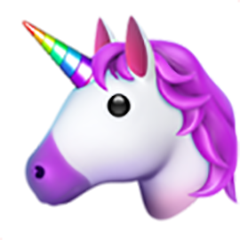
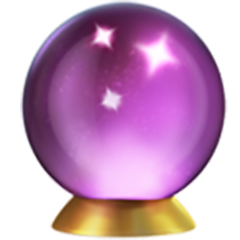
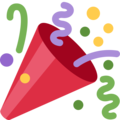
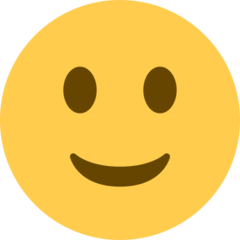
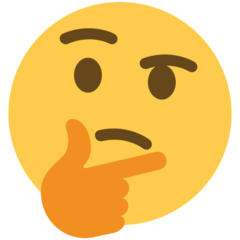
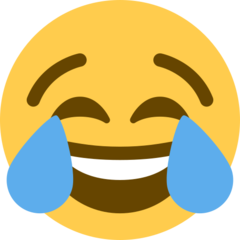
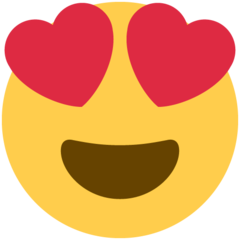
export default {
data() {
return {
}
},
methods: {
async callApi(method, url, dataObj) {
try {
return await axios({
method: method,
url: url,
data: dataObj
});
} catch (e) {
return e.response
}
}
}
}
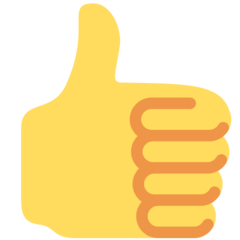
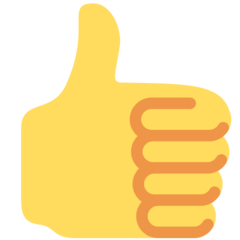
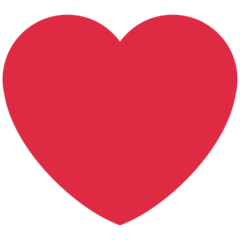
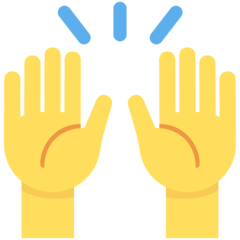
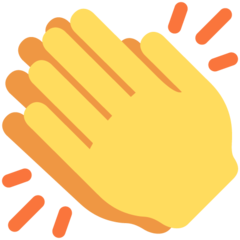
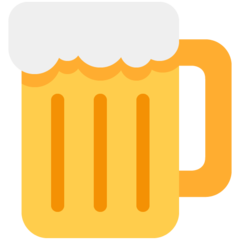
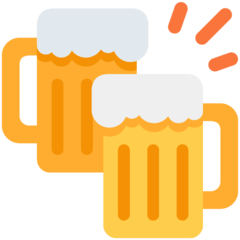
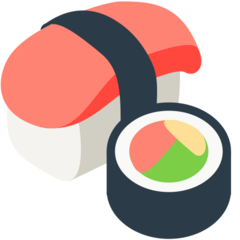
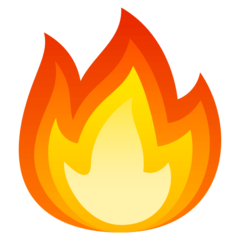
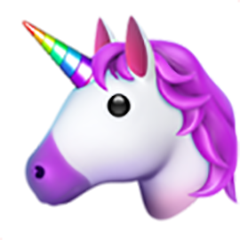
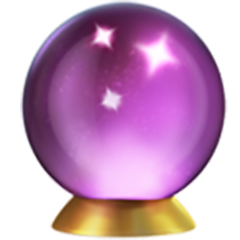
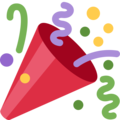
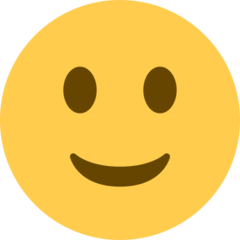
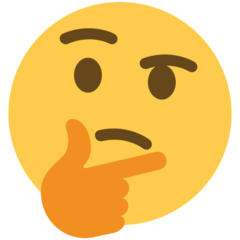
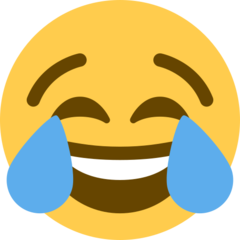
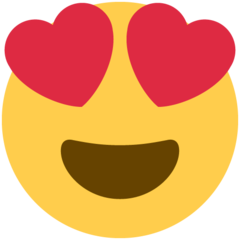