Connecting to a MySQL database in NodeJS is actually quite simple. First off, you'll need to make sure you have a local MySQL server on your machine and then you can follow these steps.
To start off you'll want to create a new folder, navigate to that folder in a shell prompt, and then install the NodeJS Mysql package:
npm i mysql
Next, create a new file called index.js inside of our new folder with the following contents:
var mysql = require('mysql');
var connection = mysql.createConnection({
host: "127.0.0.1",
user: "root",
password: ""
});
connection.connect(function(err) {
if (err) throw err;
console.log("Connected to MySQL Database");
});
Make sure that you've changed your database credentials in the createConnection object, and then run the following command in terminal:
node index.js
You should see a message output that says Connected to MySQL Database . And just like that you've successfully connected to your local MySQL server.
Next, we'll see how we can run a query to fetch some rows from a database. Go ahead and create a new database. In this example I am going to create a database with the name of nodemysql, I'm also going to create a table called users, with 2 columns. A primary id and a name column.
We'll need to add some dummy data into our new table. So, I will add the following data to my table:
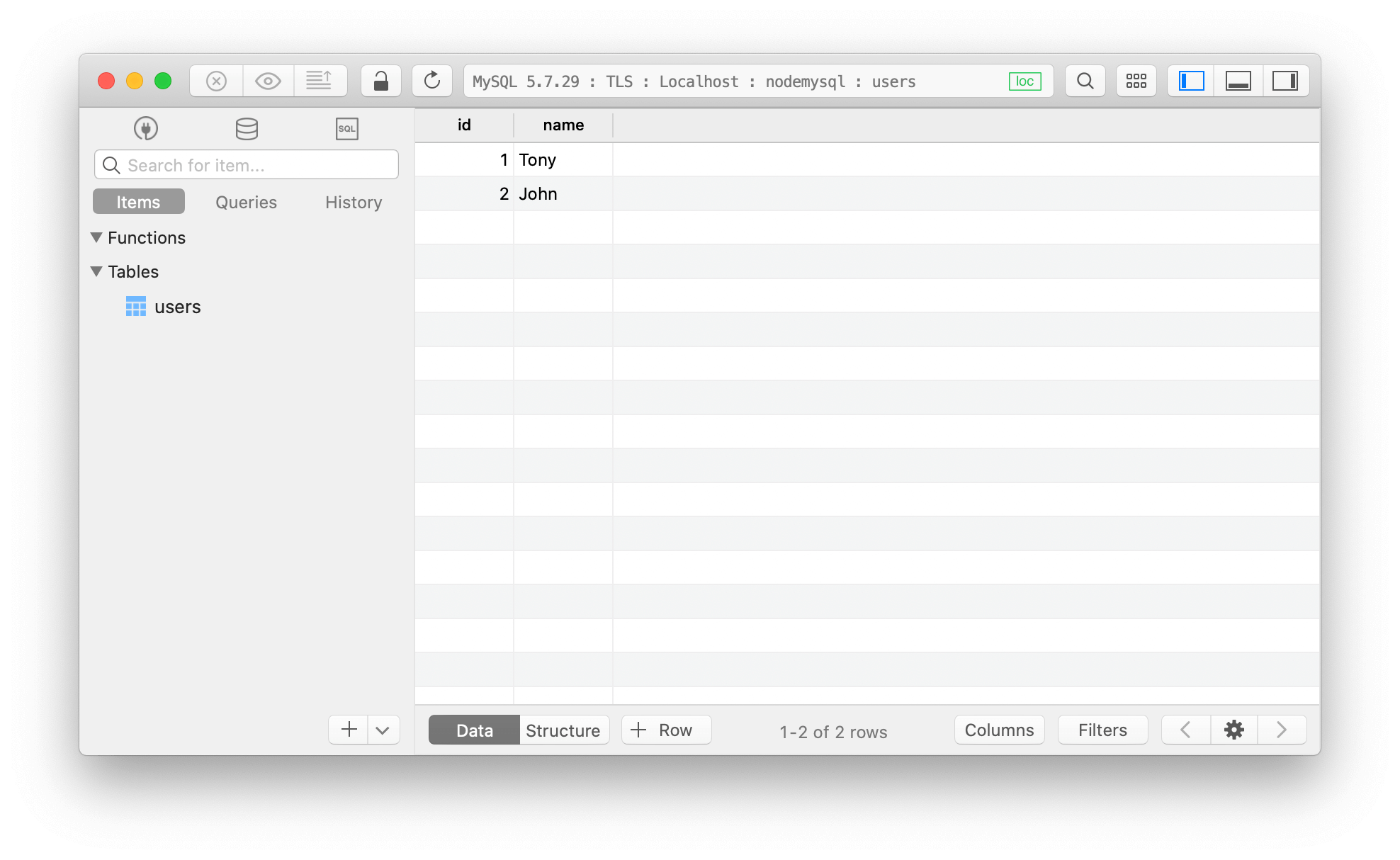
Finally, in order to connect to our database and list out our users we can modify our index.js file to look like:
var mysql = require('mysql');
var connection = mysql.createConnection({
host: "127.0.0.1",
user: "root",
password: "",
database: "nodemysql"
});
connection.connect(function(err) {
if (err) throw err;
connection.query("SELECT * FROM users", function (err, result, fields) {
if (err) throw err;
console.log(result);
});
});
Now we can run node index.js again, and we'll get the following output displaying the users inside of our database:
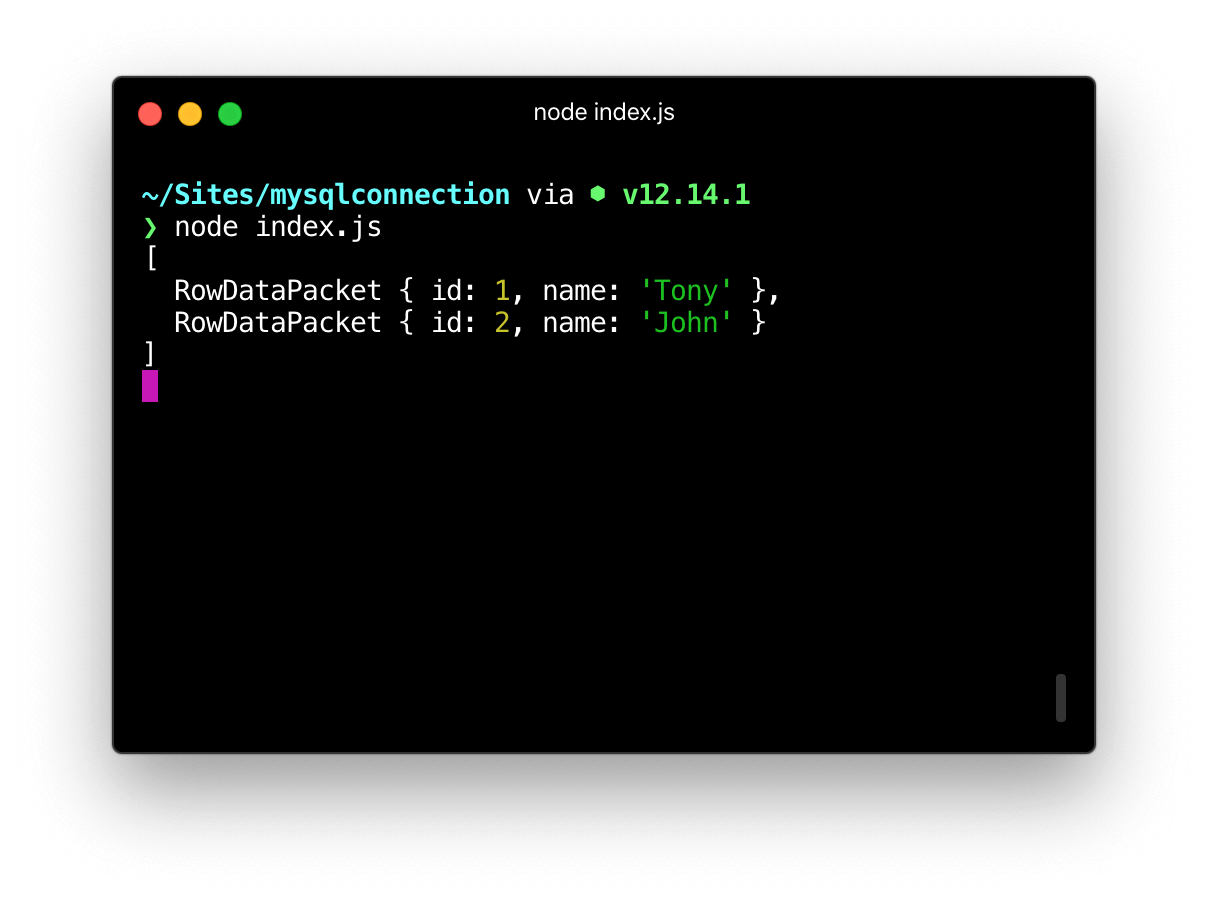
And that's the basics of creating a MySQL connection in Node JS and running a simple select query. Very simple, right? There are obviously a lot more complicated logic you will want to perform after fetching data from the database, but that's all dependent upon how you want your application to function.
Comments (0)