Livewire/AlpineJS - Confirm changing select option
Hi all! I'd like some help with this Livewire/AlpineJS scenario please:
Scenario
- I have a select dropdown with some colors.
<select wire:model="color">
<option value="red">Red</option>
<option value="blue">Blue</option>
<option value="green">Green</option>
</select>
- When the user selects an option, I'd like to show a confirm message asking if they're sure e.g.
confirm('Are you sure?')
- If they are, change the color to what they selected. If not, don't change the color.
Examples
- Color selected is yellow -> The user clicks on red -> Are you sure? -> No -> Color selected is yellow
- Color selected is yellow -> The user clicks on red -> Are you sure? -> Yes -> Color selected is red
Hi there,
Here's a solution using Livewire and AlpineJS. Livewire is used to keep the server state of the color and AlpineJS is used to handle the confirmation logic.
First, define your Livewire component. Let's call it ColorComponent:
<?php
namespace App\Http\Livewire;
use Livewire\Component;
class ColorComponent extends Component
{
public $color;
protected $listeners = ['confirmColorChange'];
public function confirmColorChange($newColor)
{
$this->color = $newColor;
}
public function render()
{
return view('livewire.color-component');
}
}
Next, create your Livewire view (color-component.blade.php
):
<div x-data="{ selectedColor: '{{ $color }}', tempColor: '' }">
<select x-model="tempColor" @change="if (confirm('Are you sure?')) { selectedColor = tempColor; $wire.emit('confirmColorChange', selectedColor); } else { tempColor = selectedColor; }">
<option value="red">Red</option>
<option value="blue">Blue</option>
<option value="green">Green</option>
</select>
<p>Color selected is <span x-text="selectedColor"></span></p>
</div>
In this solution, AlpineJS handles the local state of the selected color. When the select dropdown value changes, it prompts the user for confirmation. If the user confirms the action, the selectedColor
is set to tempColor
, and an event is emitted to the Livewire component to sync the state with the server. If the user cancels the action, the tempColor is reset back to selectedColor, effectively ignoring the attempted change.
Remember to include both Livewire and AlpineJS in your main layout:
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
...
@livewireStyles
</head>
<body>
...
@livewireScripts
<script src="https://cdn.jsdelivr.net/gh/alpinejs/[email protected]/dist/alpine.min.js" defer></script>
</body>
</html>
Let me know if you have any questions!
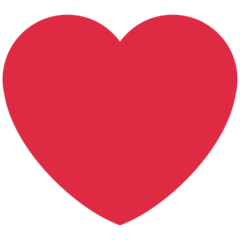
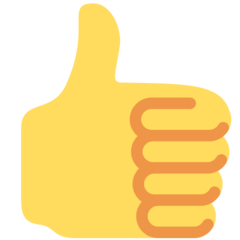
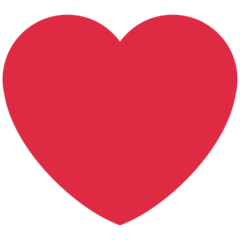
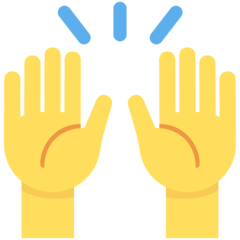
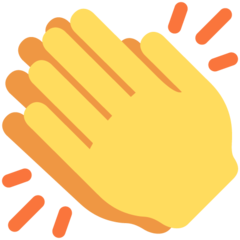
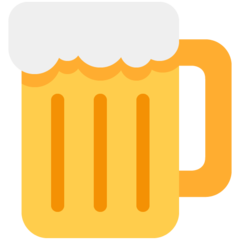
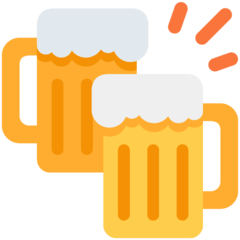
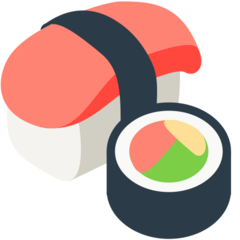
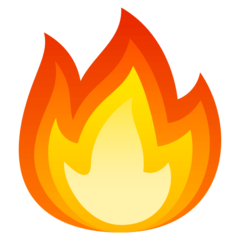
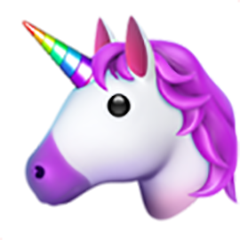
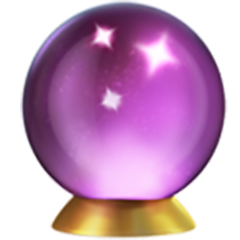
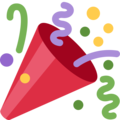
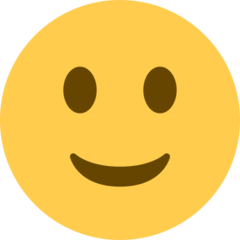
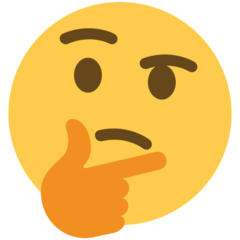
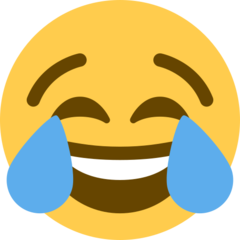
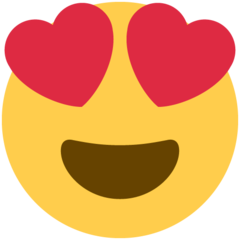
This is fantastic, thank you so much!
No problem at all! And good luck with your project!